How To Change Value In List Python
What is Python Listing?
A list is a sequence of values (similar to an assortment in other programming languages merely more versatile)
The values in a list are called items or sometimes elements.
The important properties of Python lists are as follows:
- Lists are ordered – Lists remember the social club of items inserted.
- Accessed past index – Items in a list tin be accessed using an index.
- Lists can contain whatever sort of object – Information technology can be numbers, strings, tuples and even other lists.
- Lists are changeable (mutable) – You lot can modify a list in-place, add new items, and delete or update existing items.
Create a List
There are several ways to create a new list; the simplest is to enclose the values in square brackets []
# A list of integers 50 = [1, two, 3] # A listing of strings L = ['red', 'dark-green', 'blue']
The items of a list don't have to be the same type. The following list contains an integer, a string, a bladder, a circuitous number, and a boolean.
# A list of mixed datatypes L = [ one, 'abc', 1.23, (3+4j), Truthful]
A list containing zero items is called an empty list and you lot tin can create ane with empty
brackets []
There is one more way to create a list based on existing list, called List comprehension.
The list() Constructor
You can convert other data types to lists using Python'due south list() constructor.
# Catechumen a string to a list L = list('abc') impress(L) # Prints ['a', 'b', 'c']
# Convert a tuple to a list L = list((ane, two, iii)) print(L) # Prints [1, 2, 3]
Nested List
A list can contain sublists, which in turn can contain sublists themselves, and so on. This is known every bit nested list.
You can use them to arrange data into hierarchical structures.
Fifty = ['a', ['bb', ['ccc', 'ddd'], 'ee', 'ff'], 'g', 'h']
Read more almost information technology in the nested listing tutorial.
Access List Items by Index
You tin think of a list every bit a human relationship betwixt indexes and values. This human relationship is chosen a mapping; each alphabetize maps to one of the values. The indexes for the values in a list are illustrated every bit beneath:
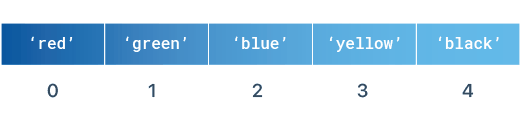
Note that the showtime chemical element of a list is e'er at index zero.
Yous can access individual items in a listing using an index in foursquare brackets.
Fifty = ['red', 'green', 'blue', 'yellow', 'blackness'] print(Fifty[0]) # Prints blood-red print(L[two]) # Prints blue
Python will raise an IndexError
error, if you utilize an index that exceeds the number of items in your list.
Fifty = ['red', 'green', 'blue', 'xanthous', 'blackness'] print(L[10]) # Triggers IndexError: listing index out of range
Negative List Indexing
You can access a list past negative indexing too. Negative indexes count backward from the end of the list. So, L[-1]
refers to the last item, L[-2]
is the 2d-concluding, and and so on.
The negative indexes for the items in a listing are illustrated as below:
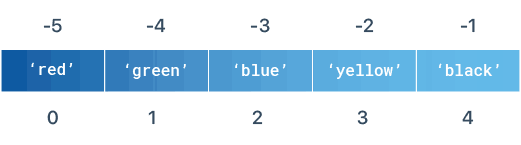
L = ['cherry', 'green', 'bluish', 'yellow', 'black'] impress(L[-i]) # Prints black impress(50[-2]) # Prints yellowish
Access Nested Listing Items
Similarly, you lot can access individual items in a nested listing using multiple indexes. The first alphabetize determines which listing to employ, and the second indicates the value within that list.
The indexes for the items in a nested list are illustrated as below:
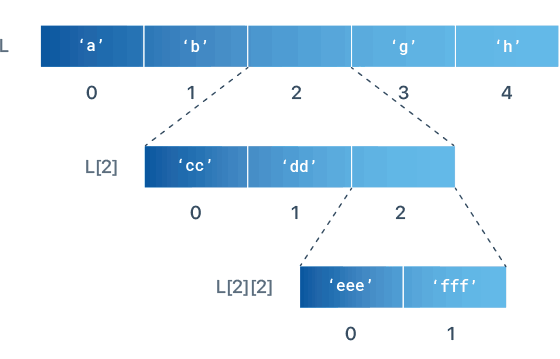
50 = ['a', 'b', ['cc', 'dd', ['eee', 'fff']], 'g', 'h'] print(L[2][2]) # Prints ['eee', 'fff'] print(L[2][2][0]) # Prints eee
Slicing a Listing
A segment of a list is called a piece and you tin can extract one by using a slice operator. A slice of a list is also a list.
The slice operator [n:grand] returns the function of the list from the "n-th" item to the "m-th" particular, including the first simply excluding the last.
50 = ['a', 'b', 'c', 'd', 'e', 'f'] print(Fifty[2:v]) # Prints ['c', 'd', 'e'] impress(L[0:ii]) # Prints ['a', 'b'] print(L[three:-1]) # Prints ['d', 'due east']
Read more about information technology in the list slicing tutorial.
Change Item Value
Yous can replace an existing chemical element with a new value past assigning the new value to the index.
L = ['blood-red', 'dark-green', 'bluish'] Fifty[0] = 'orangish' print(L) # Prints ['orange', 'dark-green', 'blueish'] L[-1] = 'violet' print(L) # Prints ['orangish', 'green', 'violet']
Add items to a listing
To add together new values to a listing, apply append() method. This method adds items only to the end of the listing.
L = ['red', 'green', 'yellow'] Fifty.append('blue') print(50) # Prints ['crimson', 'greenish', 'yellow', 'blue']
If you desire to insert an particular at a specific position in a list, use insert() method. Note that all of the values in the listing after the inserted value will be moved downward one index.
L = ['red', 'dark-green', 'yellowish'] L.insert(1,'blue') print(Fifty) # Prints ['red', 'blue', 'green', 'yellow']
Combine Lists
You can merge i list into another by using extend() method. Information technology takes a list as an argument and appends all of the elements.
L = ['red', 'green', 'yellow'] L.extend([1,2,3]) impress(L) # Prints ['red', 'green', 'yellow', ane, 2, three]
Alternatively, you can utilize the concatenation operator +
or the augmented assignment operator +=
# concatenation operator 50 = ['red', 'green', 'blue'] L = Fifty + [1,2,3] impress(L) # Prints ['red', 'greenish', 'blue', one, 2, 3] # augmented assignment operator Fifty = ['red', 'dark-green', 'blue'] L += [ane,two,3] print(L) # Prints ['red', 'greenish', 'blue', ane, ii, 3]
Remove items from a list
At that place are several ways to remove items from a list.
Remove an Item by Index
If you know the index of the item y'all want, you lot can apply pop() method. It modifies the listing and returns the removed detail.
If no index is specified, pop()
removes and returns the last item in the list.
L = ['ruby', 'green', 'blue'] x = L.popular(1) print(Fifty) # Prints ['carmine', 'blue'] # removed detail print(10) # Prints green
If yous don't demand the removed value, employ the del statement.
L = ['carmine', 'green', 'blueish'] del Fifty[1] print(L) # Prints ['reddish', 'blueish']
Remove an Item by Value
If you're not sure where the detail is in the listing, use remove() method to delete it by value.
L = ['red', 'green', 'blue'] Fifty.remove('carmine') print(L) # Prints ['dark-green', 'blue']
Merely keep in listen that if more one instance of the given item is present in the list, so this method removes merely the beginning instance.
L = ['red', 'green', 'blue', 'red'] 50.remove('red') impress(L) # Prints ['greenish', 'bluish', 'crimson']
Remove Multiple Items
To remove more than one items, use the del keyword with a piece alphabetize.
L = ['ruddy', 'green', 'bluish', 'xanthous', 'black'] del L[one:iv] print(Fifty) # Prints ['red', 'black']
Remove all Items
Use clear() method to remove all items from the list.
Fifty = ['reddish', 'green', 'blue'] L.articulate() impress(L) # Prints []
Listing Replication
The replication operator *
repeats a listing a given number of times.
50 = ['red'] L = 50 * three impress(L) # Prints ['reddish', 'carmine', 'reddish']
Find List Length
To find the number of items in a list, use len() method.
L = ['red', 'greenish', 'blue'] print(len(L)) # Prints 3
Check if particular exists in a listing
To determine whether a value is or isn't in a list, you can use in and not in operators with if statement.
# Check for presence L = ['cherry-red', 'green', 'blue'] if 'cerise' in L: print('yep') # Check for absenteeism L = ['red', 'green', 'bluish'] if 'xanthous' not in L: print('yes')
Iterate through a Listing
The most mutual mode to iterate through a list is with a for loop.
50 = ['red', 'light-green', 'blueish'] for detail in 50: print(item) # Prints red # Prints green # Prints blue
This works well if yous only demand to read the items of the list. But if you want to update them, you lot need the indexes. A common style to practice that is to combine the range()
and len()
functions.
# Loop through the list and double each detail L = [1, 2, 3, 4] for i in range(len(L)): L[i] = Fifty[i] * ii impress(Fifty) # Prints [2, 4, 6, 8]
Python List Methods
Python has a set of congenital-in methods that y'all tin telephone call on list objects.
Method | Description |
append() | Adds an item to the end of the list |
insert() | Inserts an item at a given position |
extend() | Extends the listing by appending all the items from the iterable |
remove() | Removes showtime instance of the specified detail |
pop() | Removes the item at the given position in the listing |
clear() | Removes all items from the list |
copy() | Returns a shallow re-create of the list |
count() | Returns the count of specified particular in the list |
index() | Returns the index of first instance of the specified item |
reverse() | Reverses the items of the listing in place |
sort() | Sorts the items of the list in place |
Congenital-in Functions with Listing
Python also has a set of built-in functions that you tin use with listing objects.
Method | Description |
all() | Returns True if all list items are true |
any() | Returns Truthful if any listing item is true |
enumerate() | Takes a list and returns an enumerate object |
len() | Returns the number of items in the list |
list() | Converts an iterable (tuple, string, set etc.) to a list |
max() | Returns the largest item of the listing |
min() | Returns the smallest item of the listing |
sorted() | Returns a sorted list |
sum() | Sums items of the list |
Source: https://www.learnbyexample.org/python-list/
Posted by: millerwervaing.blogspot.com
0 Response to "How To Change Value In List Python"
Post a Comment